Best Practices
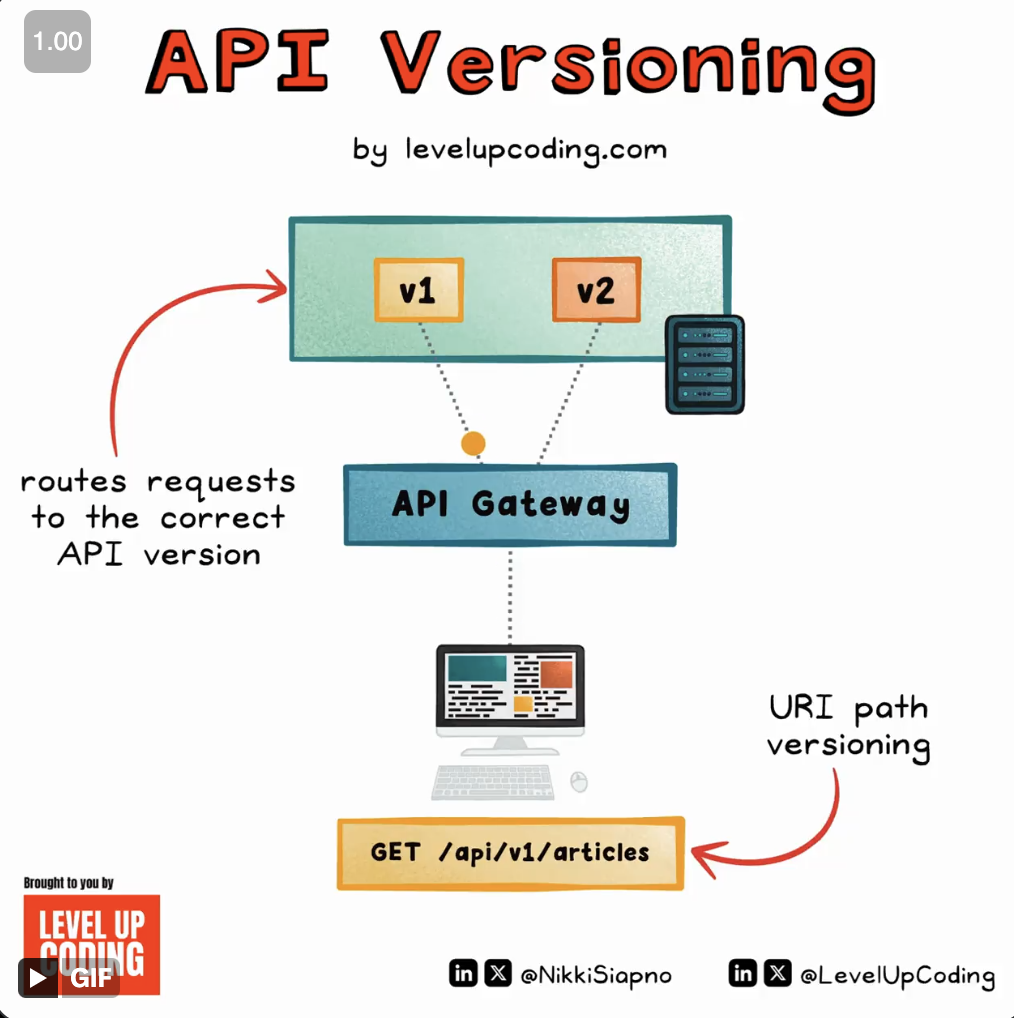
Tools
- API Mocks for Free - https://designer.mocky.io/
- Postman
- Insomnia
- Bruno
- REST Client VSCode Extension
- Mintilify - Modern Documentation
API Architectures Styles
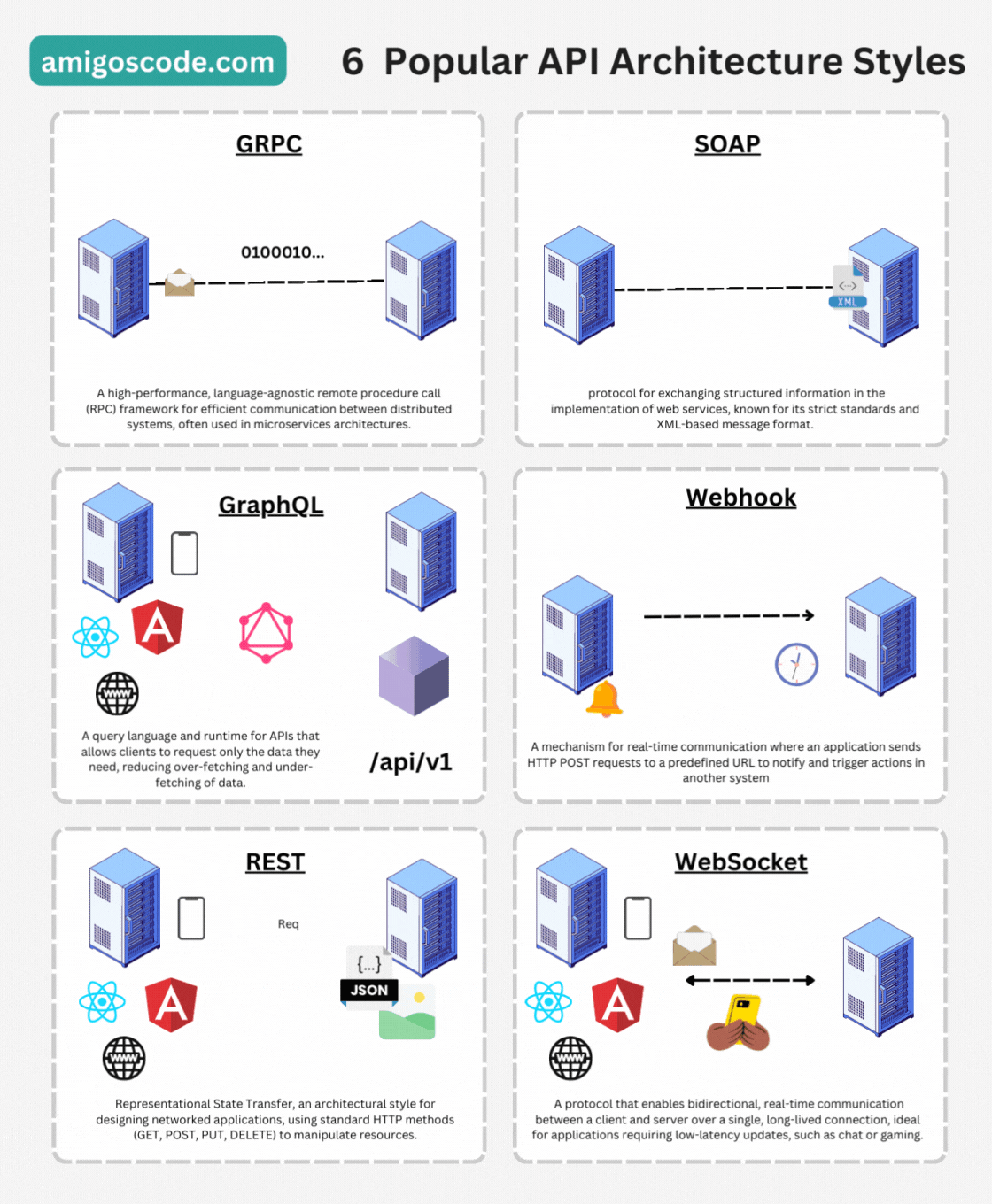
HTTP - Hypertext Transfer Protocol
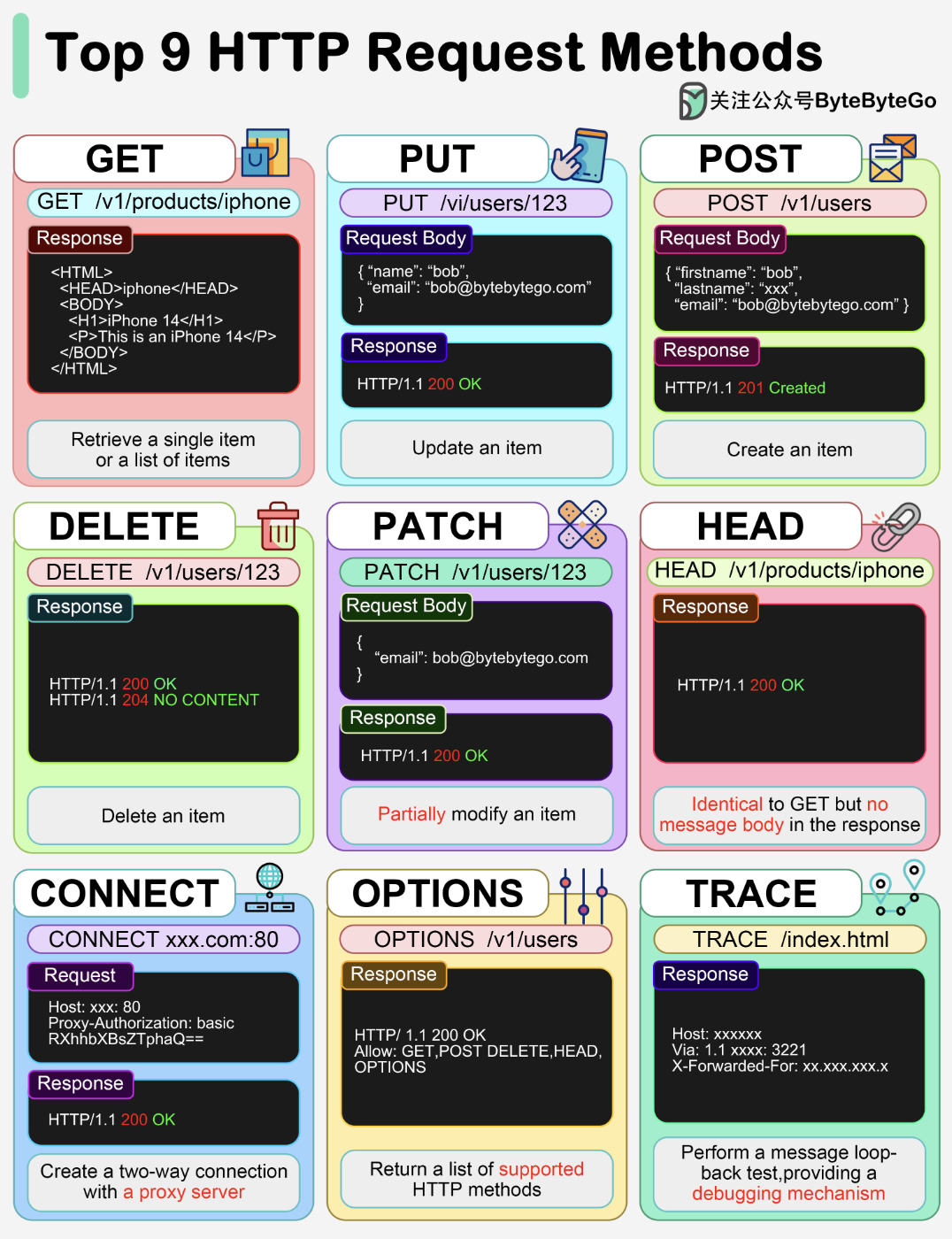
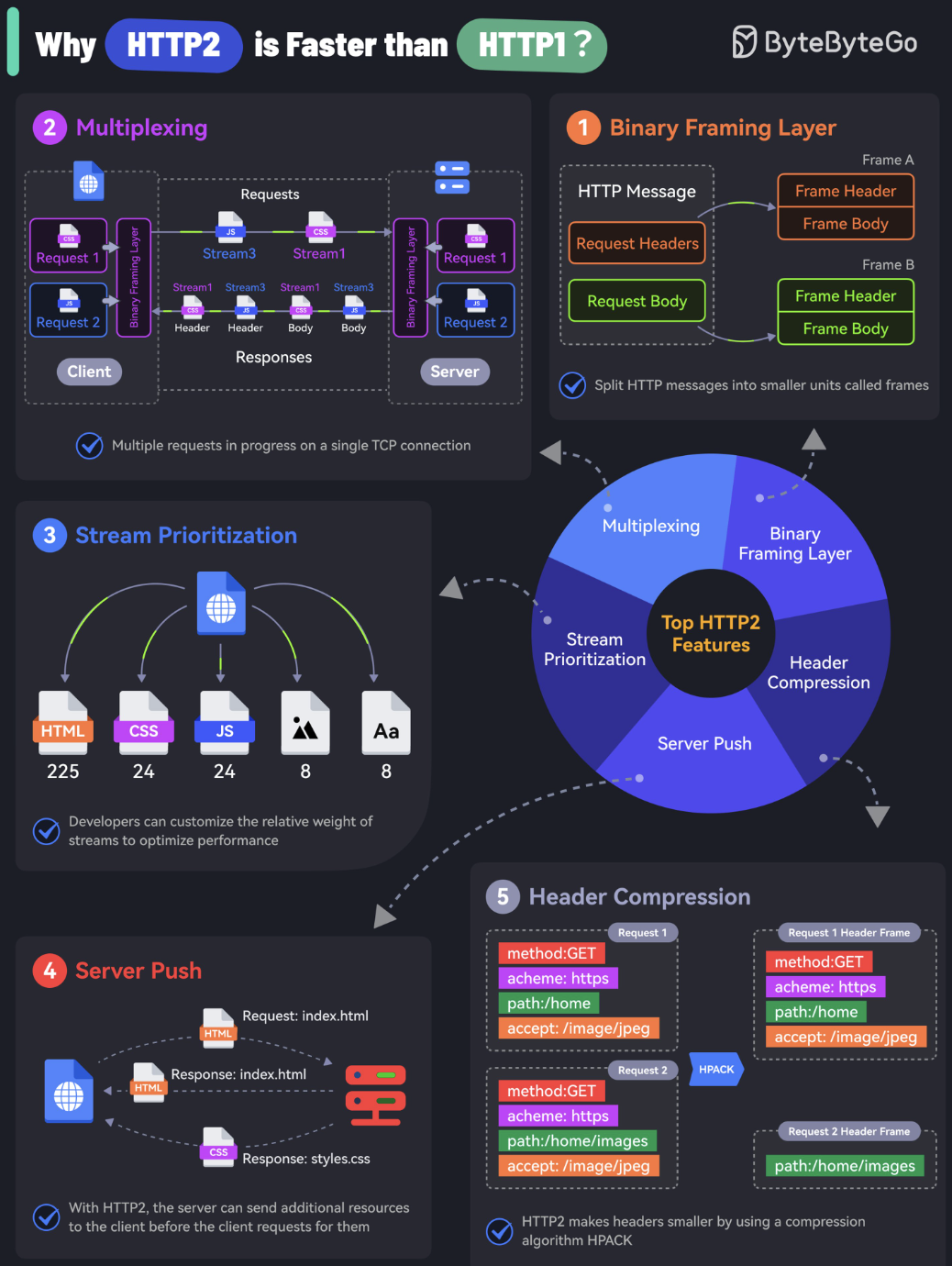
Performance
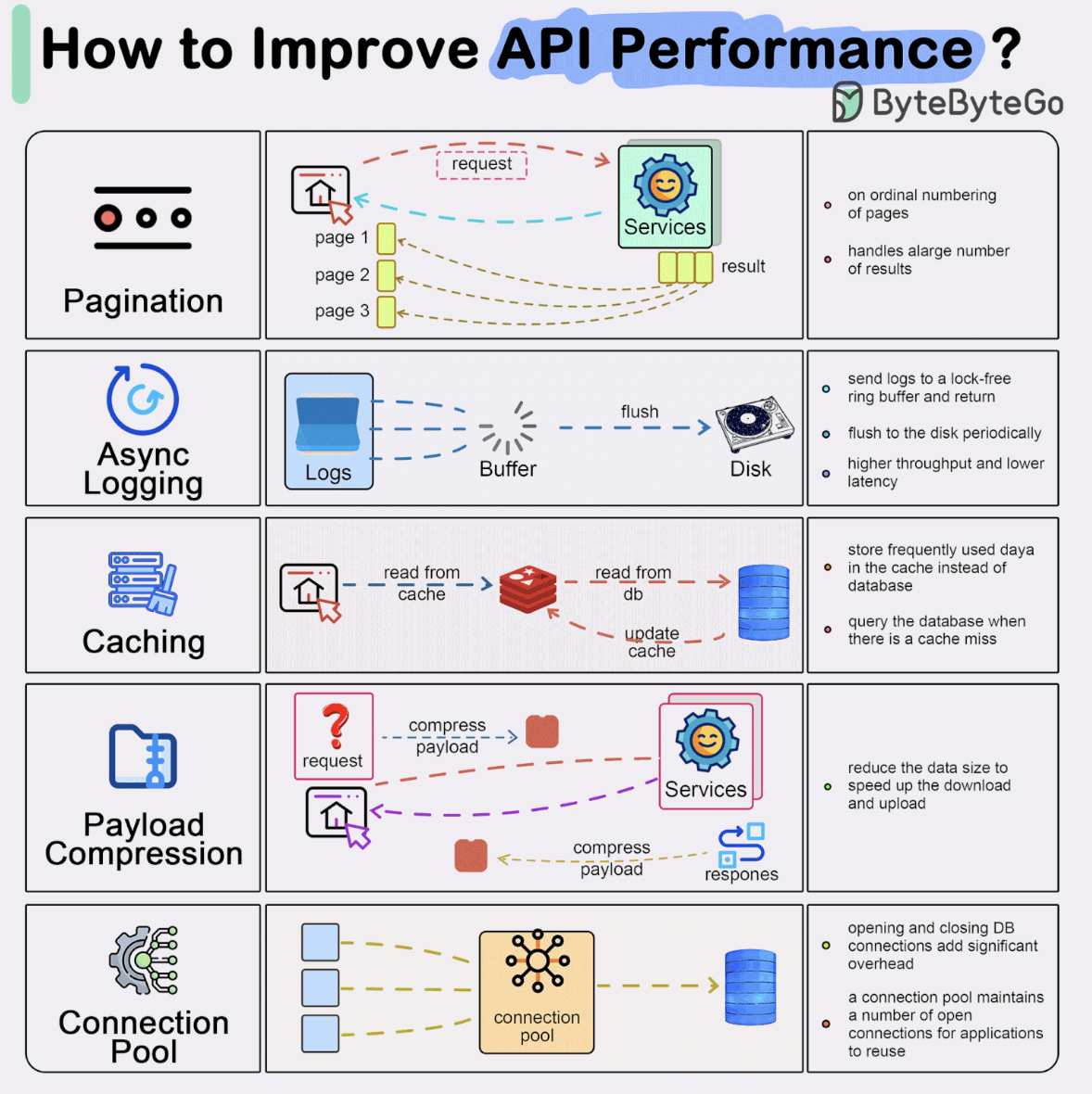
HTTP Haders
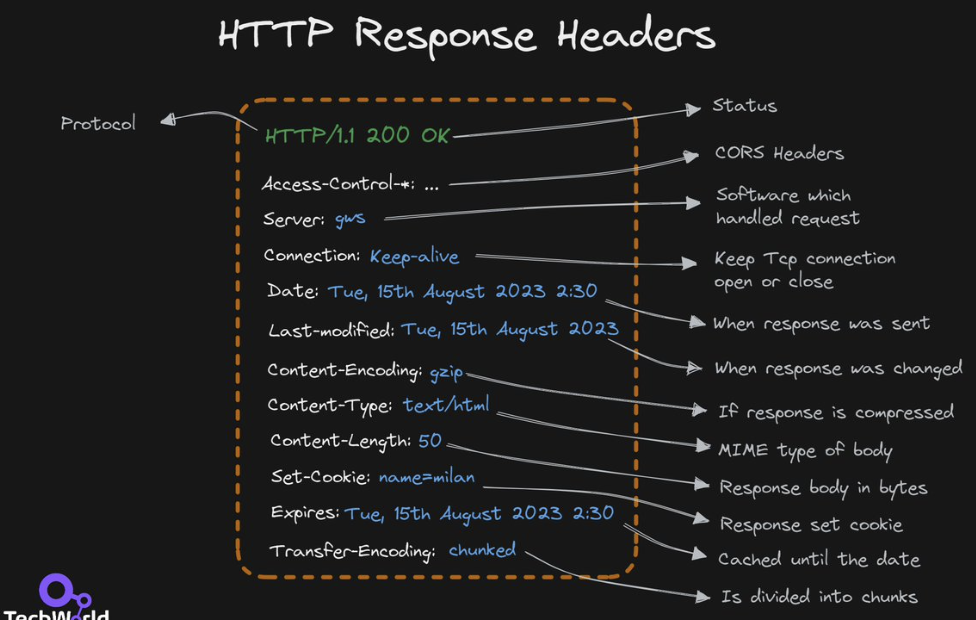
HTTP Status Codes
Informational 1xx
- 100 - Continue - Client should continue with request.
- 101 - Switching Protocols - Server is switching protocols.
- 102 - Processing - Server has received and is processing the request.
- 103 - Processing - Server has received and is processing the request.
- 122 - Request-uri too long - URI is longer than a maximum of 2083 characters.
Success 2xx
These codes indicate success. The body section if present is the object returned by the request. It is a MIME format object. It is in MIME format, and may only be in text/plain, text/html or one fo the formats specified as acceptable in the request.
- 200 - Ok - The request was fulfilled.
- 201 - Created - Following a POST command, this indicates success, but the textual part of the response line indicates the URI by which the newly created document should be known.
- 202 - Accepted - The request has been accepted for processing, but the processing has not been completed. The request may or may not eventually be acted upon, as it may be disallowed when processing actually takes place. there is no facility for status returns from asynchronous operations such as this.
- 203 - Partial Information - When received in the response to a GET command, this indicates that the returned metainformation is not a definitive set of the object from a server with a copy of the object, but is from a private overlaid web. This may include annotation information about the object, for example.
- 204 - No Response - Server has received the request but there is no information to send back, and the client should stay in the same document view. This is mainly to allow input for scripts without changing the document at the same time.
- 205 - Reset Content - Request processed, no content returned, reset document view.
- 206 - Partial Content - partial resource return due to request header.
- 207 - Multi-Status - XML, can contain multiple separate responses.
- 208 - Already Reported - results previously returned.
- 226 - Im Used - request fulfilled, reponse is instance-manipulations.
Redirection 3xx
The codes in this section indicate action to be taken (normally automatically) by the client in order to fulfill the request.
- 301 - Moved - The data requested has been assigned a new URI, the change is permanent. (N.B. this is an optimisation, which must, pragmatically, be included in this definition. Browsers with link editing capabiliy should automatically relink to the new reference, where possible)
- 302 - Found - The data requested actually resides under a different URL, however, the redirection may be altered on occasion (when making links to these kinds of document, the browser should default to using the Udi of the redirection document, but have the option of linking to the final document) as for “Forward”.
- 303 - Method - Like the found response, this suggests that the client go try another network address. In this case, a different method may be used too, rather than GET.
- 304 - Not Modified - If the client has done a conditional GET and access is allowed, but the document has not been modified since the date and time specified in If-Modified-Since field, the server responds with a 304 status code and does not send the document body to the client.
- 305 - Use Proxy - Content located elsewhere, retrieve from there.
- 306 - Switch Proxy - Subsequent requests should use the specified proxy.
- 307 - Temporary Redirect - Connect again to different URI as provided.
- 308 - Permanent Redirect - Connect again to a different URI using the same method.
Client side errors 4xx
The 4xx codes are intended for cases in which the client seems to have erred, and the 5xx codes for the cases in which the server is aware that the server has erred. It is impossible to distinguish these cases in general, so the difference is only informational.
The body section may contain a document describing the error in human readable form. The document is in MIME format, and may only be in text/plain, text/html or one for the formats specified as acceptable in the request.
- 400 - Bad Request - The request had bad syntax or was inherently impossible to be satisfied.
- 401 - Unauthorized - The parameter to this message gives a specification of authorization schemes which are acceptable. The client should retry the request with a suitable Authorization header.
- 402 - Payment Required - The parameter to this message gives a specification of charging schemes acceptable. The client may retry the request with a suitable ChargeTo header.
- 403 - Forbidden - The request is for something forbidden. Authorization will not help.
- 404 - Not Found - The server has not found anything matching the URI given.
- 405 - Method Not Allowed - Request method not supported by that resource.
- 406 - Not Acceptable - Content not acceptable according to the Accept headers.
- 407 - Proxy Authentication Required - Client must first authenticate itself with the proxy.
- 408 - Request Timeout - Server timed out waiting for the request.
- 409 - Conflict - Request could not be processed because of conflict.
- 410 - Gone - Resource is no longer available and will not be available again.
- 411 - Length Required - Request did not specify the length of its content.
- 412 - Precondition Failed - Server does not meet request preconditions.
- 413 - Request Entity Too Large - Request is larger than the server is willing or able to process.
- 414 - Request URI Too Large - URI provided was too long for the server to process.
- 415 - Unsupported Media Type - Server does not support media type.
- 416 - Requested Rage Not Satisfiable - Client has asked for unprovidable portion of the file.
- 417 - Expectation Failed - Server cannot meet requirements of Expect request-header field.
- 418 - I’m a teapot - I’m a teapot.
- 420 - Enhance Your Calm - Twitter rate limiting.
- 421 - Misdirected Request - Server is not able to produce a response.
- 422 - Unprocessable Entity - Request unable to be followed due to semantic errors.
- 423 - Locked - Resource that is being accessed is locked.
- 424 - Failed Dependency - Request failed due to failure of a previous request.
- 426 - Upgrade Required - Client should switch to a different protocol.
- 428 - Precondition Required - Origin server requires the request to be conditional.
- 429 - Too Many Requests - User has sent too many requests in a given amount of time.
- 431 - Request Header Fields Too Large - Server is unwilling to process the request.
- 444 - No Response - Server returns no information and closes the connection.
- 449 - Retry With - Request should be retried after performing action.
- 450 - Blocked By Windows Parental Controls - Windows Parental Controls blocking access to webpage.
- 451 - Wrong Exchange Server - The server cannot reach the client’s mailbox.
- 499 - Client Closed Request - Connection closed by client while HTTP server is processing.
Server side error 5xx
This means that even though the request appeared to be valid something went wrong at the server level and it wasn’t able to return anything.
- 500 - Internal Error - The server encountered an unexpected condition which prevented it from fulfilling the request.
- 501 - Not Implemented - The server does not support the facility required.
- 502 - Service temporarily overloaded - The server cannot process the request due to a high load (whether HTTP servicing or other requests). The implication is that this is a temporary condition which maybe alleviated at other times.
- 503 - Gateway timeout - This is equivalent to Internal Error 500, but in the case of a server which is in turn accessing some other service, this indicates that the respose from the other service did not return within a time that the gateway was prepared to wait. As from the point of view of the clientand the HTTP transaction the other service is hidden within the server, this maybe treated identically to Internal error 500, but has more diagnostic value.
- 504 - Gateway Timeout - Gateway did not receive response from upstream server.
- 505 - Http Version Not Supported - Server does not support the HTTP protocol version.
- 506 - Variant Also Negotiates - Content negotiation for the request results in a circular reference.
- 507 - Insufficient Storage - Server is unable to store the representation.
- 508 - Loop Detected - Server detected an infinite loop while processing the request.
- 509 - Bandwidth Limit Exceeded - Bandwidth limit exceeded.
- 510 - Not Extended - Further extensions to the request are required.
- 511 - Network Authentication Required - Client needs to authenticate to gain network access.
- 598 - Network Read Timeout Error - Network read timeout behind the proxy.
- 599 - Network Connect Timeout Error - Network connect timeout behind the proxy.
REST - Representational State Transfer
-
What Is
- A REST API (also called a RESTful API or RESTful web API) is an application programming interface (API) that follows the design principles of the representational state transfer (REST) architectural style.
- REST APIs provide a flexible and lightweight way to integrate applications and connect components in microservices architectures.
- First defined in 2000 by computer scientist Dr. Roy Fielding in his doctoral dissertation, REST offers a relatively high level of flexibility, scalability, and efficiency for developers. For these reasons, REST APIs have become a common method for connecting components and applications in a microservices architecture.
-
How REST APIs Work
- REST APIs communicate using HTTP requests to perform standard database functions such as creating, reading, updating, and deleting records (also known as CRUD) on a resource.
- For example, a REST API would use a GET request to retrieve a record. A POST request creates a new record. A PUT request updates a record, and a DELETE request deletes a record. All HTTP methods can be used in API calls. A well-designed REST API is similar to a website running in a web browser with HTTP functionality built in.
- The state of a resource at a given instant, or timestamp, is known as the resource representation. This information can be delivered to a client in virtually any format, including JavaScript Object Notation (JSON), HTML, XLT, Python, PHP, or plain text. JSON is popular because it is both human- and machine-readable—and is programming language independent.
- Request headers and parameters are also important in REST API calls, as they include important identifying information such as metadata, authorizations, Uniform Resource Identifiers (URIs), cache, cookies, and more. Request and response headers, along with conventional HTTP status codes, are used in well-designed REST APIs.
-
REST API Best Practices
- While flexibility is a major advantage of REST API design, this same flexibility makes it easy to design an API that is problematic or performs poorly. For this reason, professional developers share best practices in REST API specifications.
- The OpenAPI Specification (OAS) establishes an interface for describing an API in a way that any developer or application can discover it and fully understand its parameters and capabilities. This information includes available endpoints, operations allowed on each endpoint, operation parameters, authentication methods, and more. The latest version, OAS3, includes practical tools such as the OpenAPI Generator for generating API clients and server stubs in different programming languages.
- Securing a REST API also starts with industry best practices. Use hashing algorithms for password security and HTTPS for secure data transmission. An authorization framework like OAuth 2.0 can help limit the privileges of third-party applications.
- Using a timestamp in the HTTP header, an API can also reject any request that arrives after a certain amount of time. Parameter validation and JSON Web Tokens are other ways to ensure that only authorized clients can access the API.
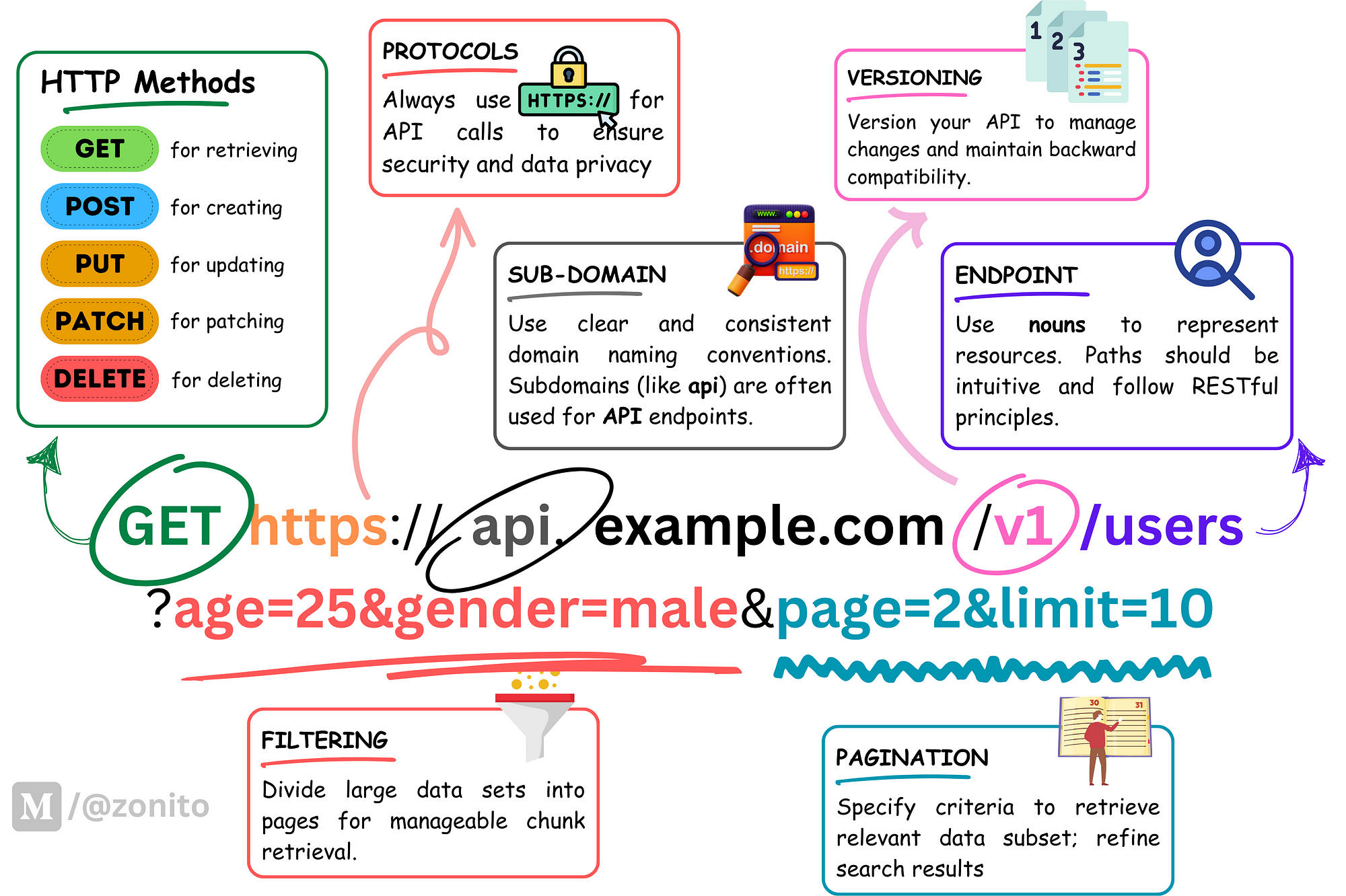
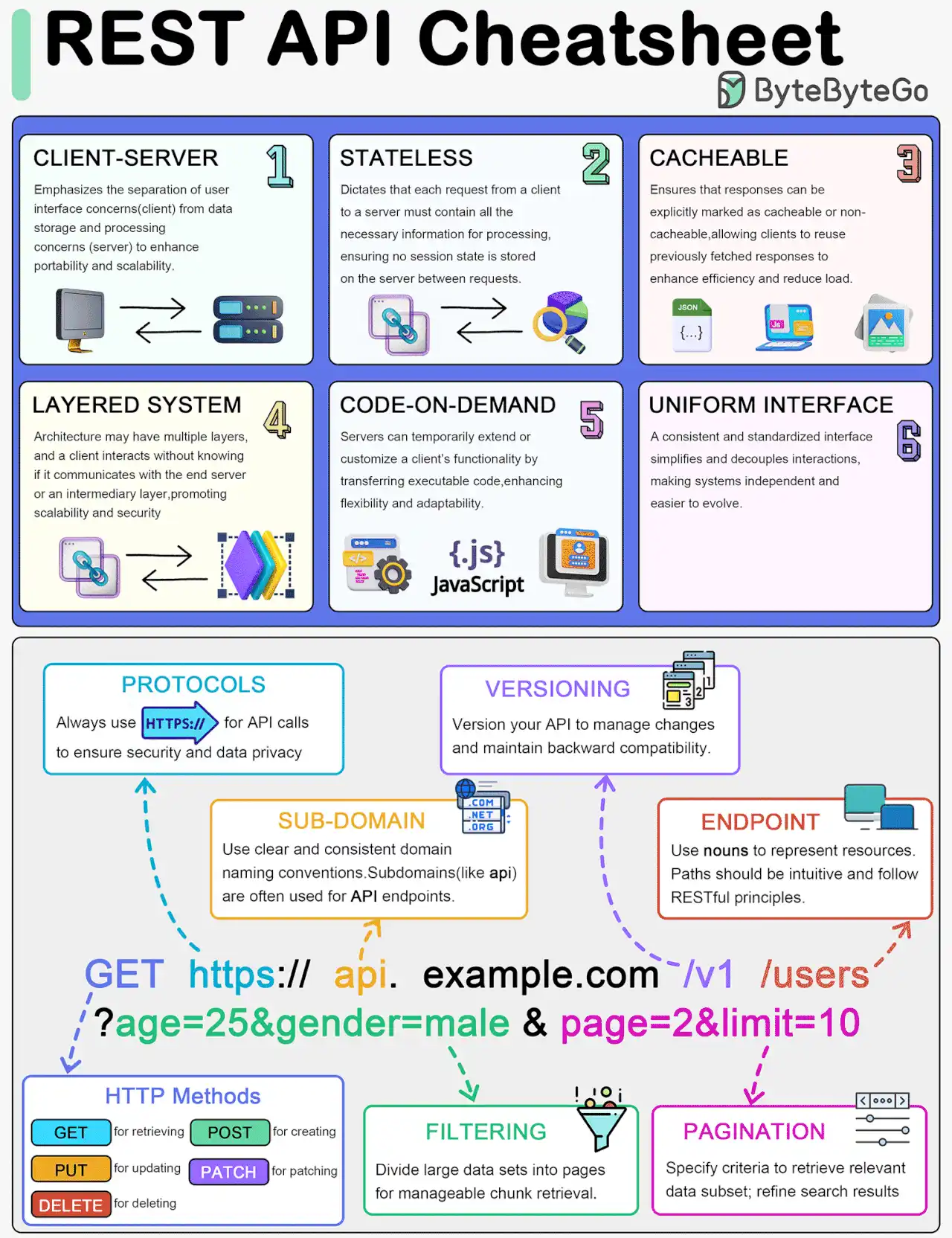
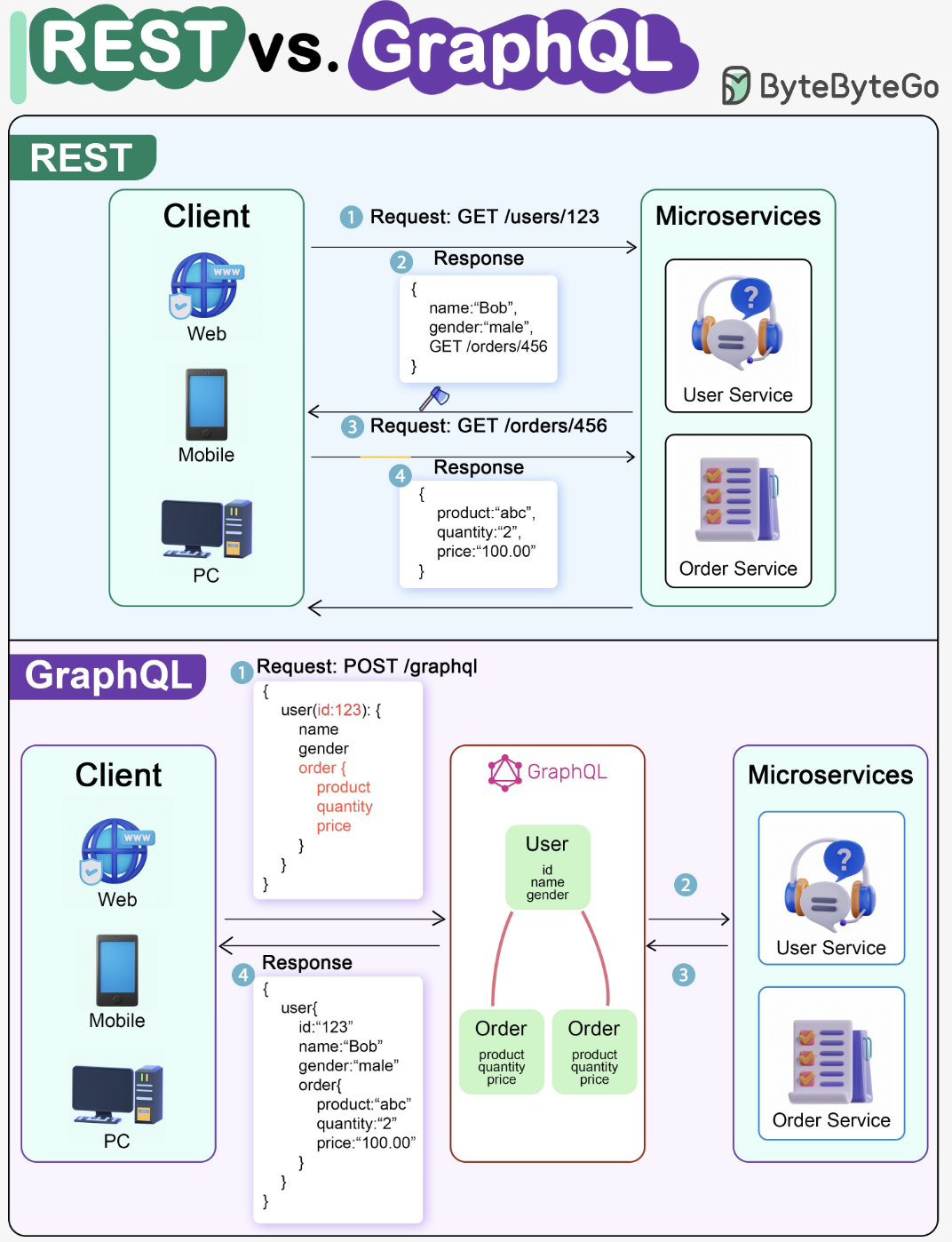
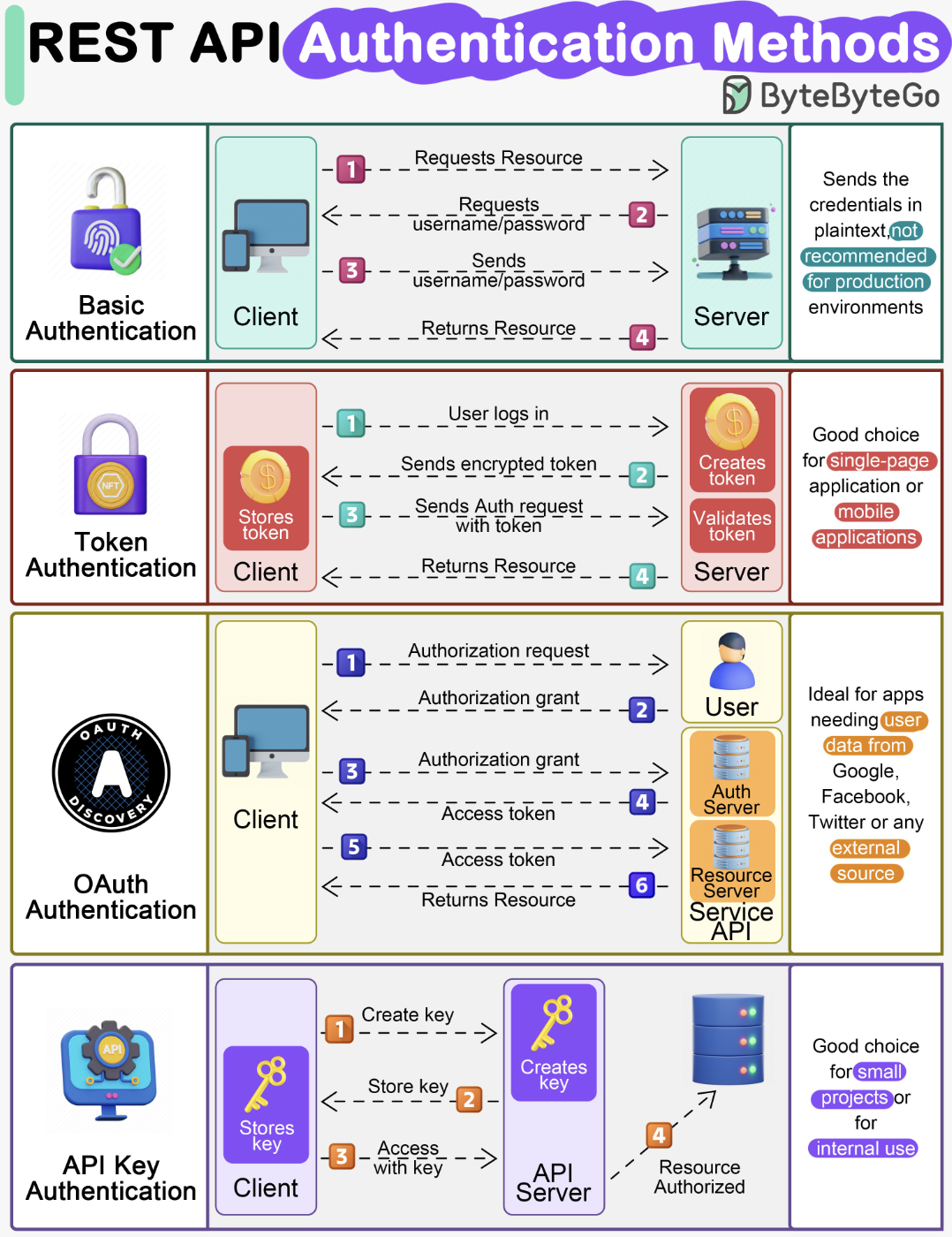
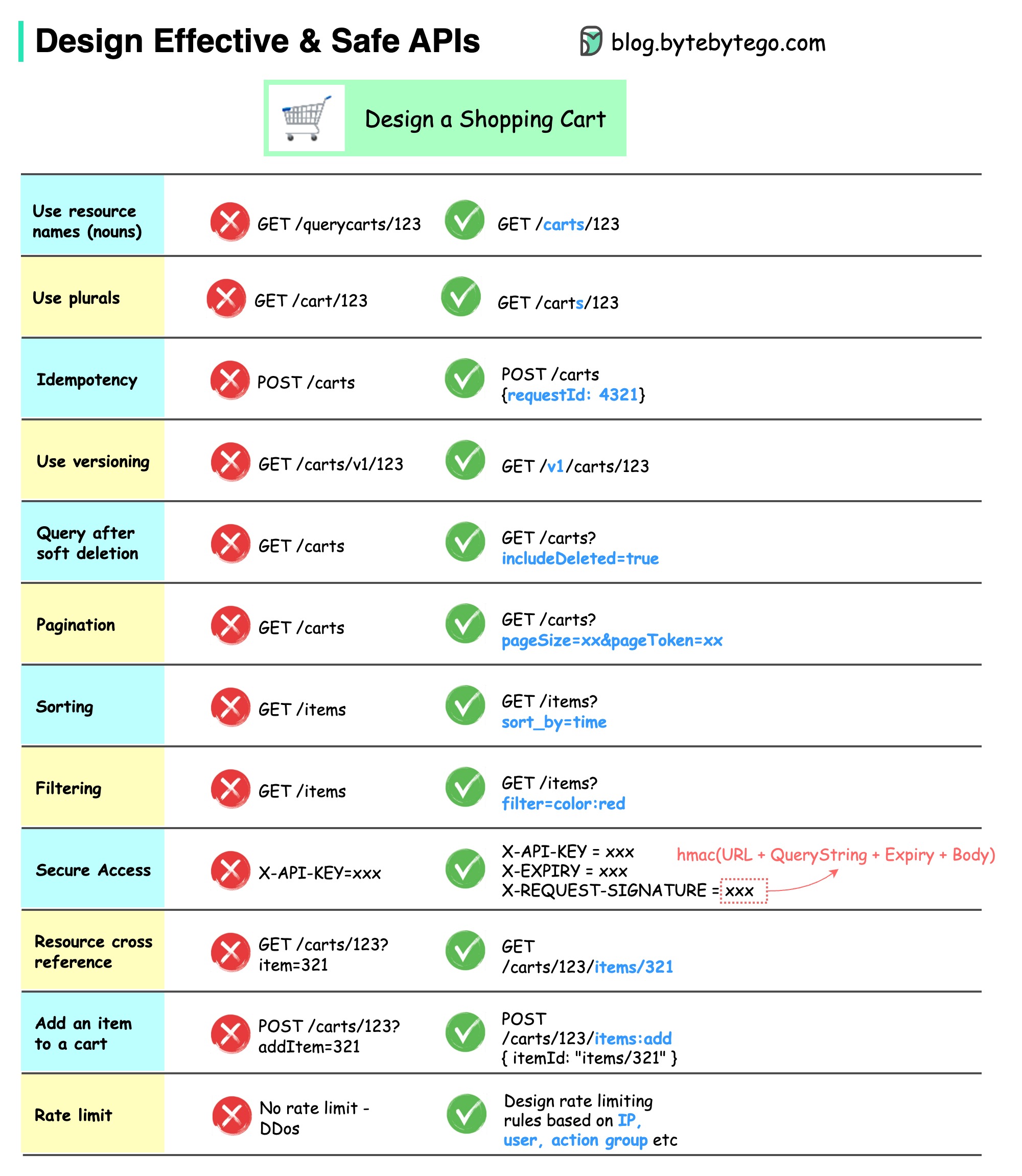
SSO - Single Sign On
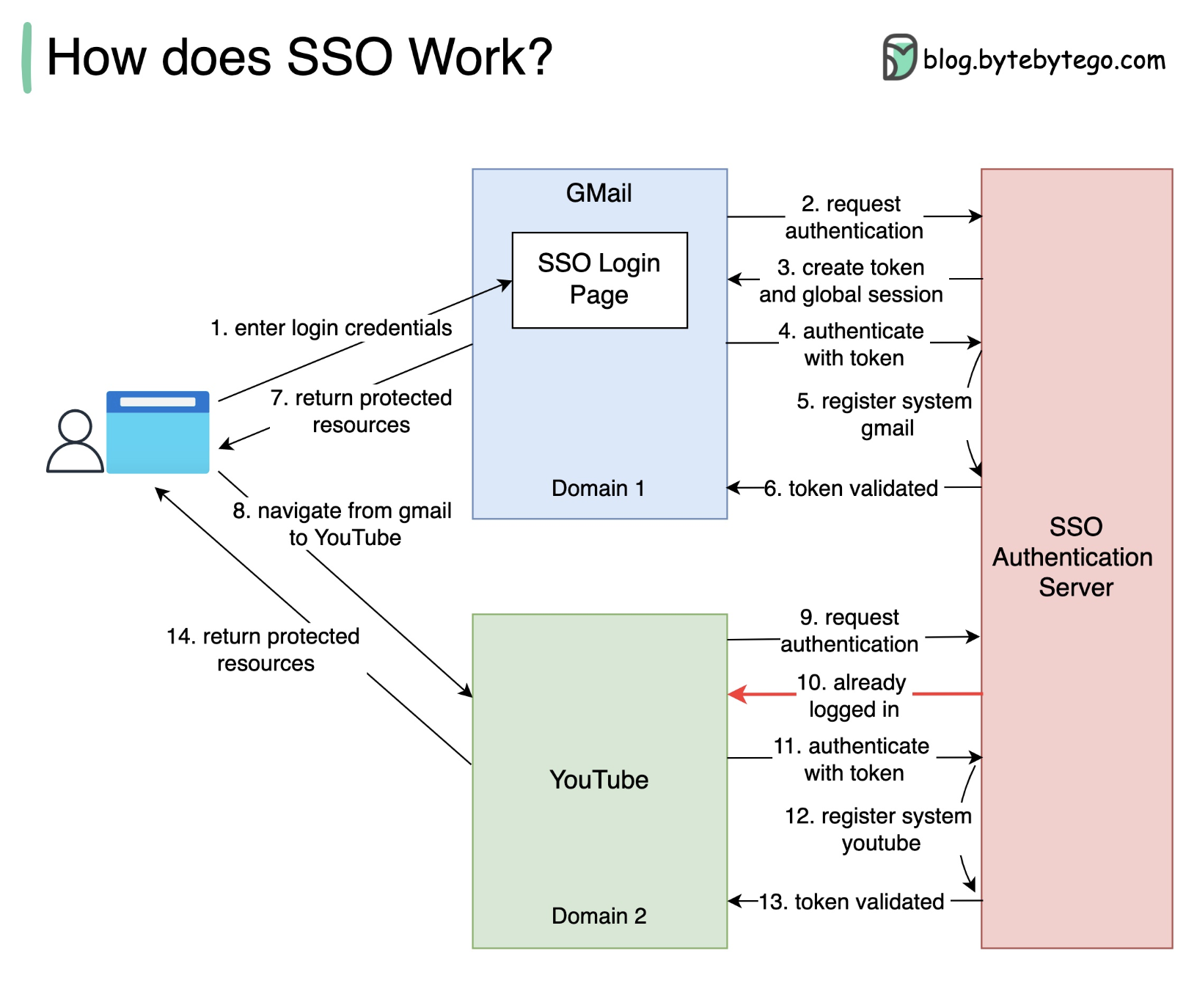
WebHooks
- Are a way for systems to communicate automatically and in real time.
- Imagine you have two applications, A and B.
- When something specific happens in application A (like a new message or a new order), it needs to notify application B about it immediately.
- Instead of application B constantly asking if something new happens (which would be inefficient), application A sends an alert to application B as soon as the event happens.
- This alert is the webhook.
- Here’s a simple summary:
- Event in application A: Something important happens (e.g. new message).
- Automatic notification: Application A sends a message (webhook) to application B.
- Response from application B: Application B receives the message and can react immediately (e.g. show the new message to the user).
- So a webhook is like an automatic notification between applications, saying “hey, something happened here, do something now”.
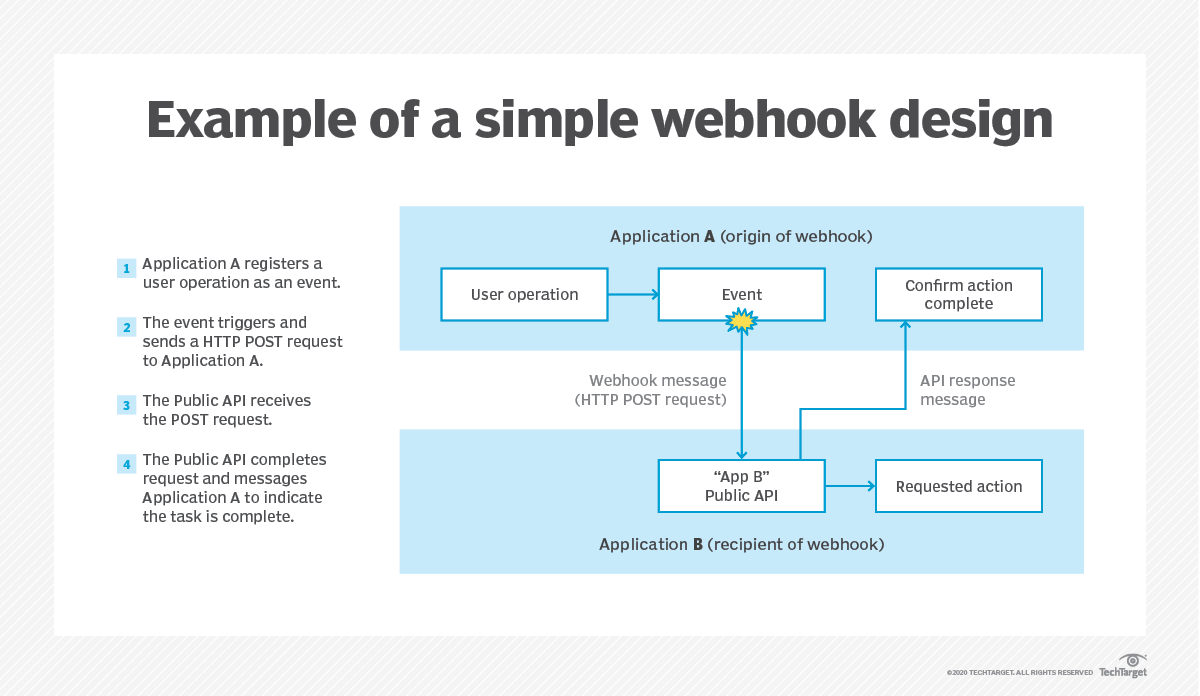
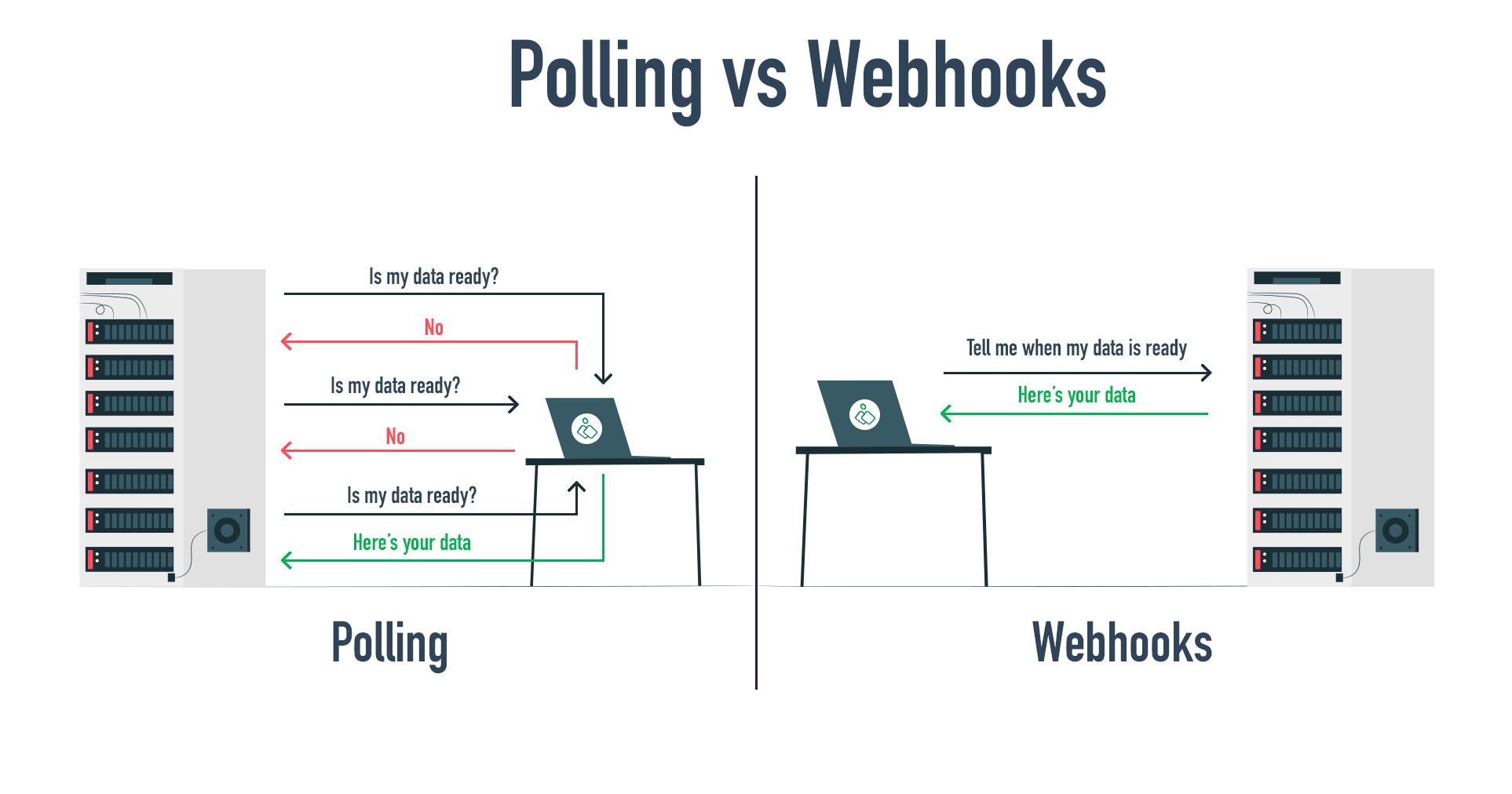
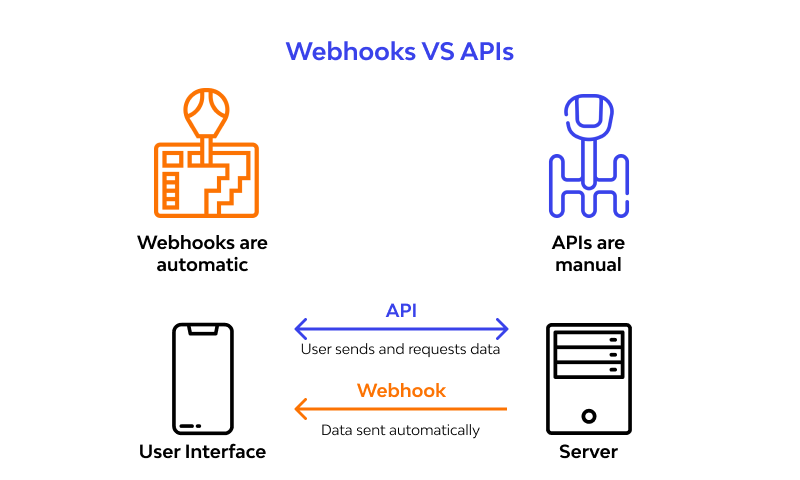
JSON Web Tokens - JWT
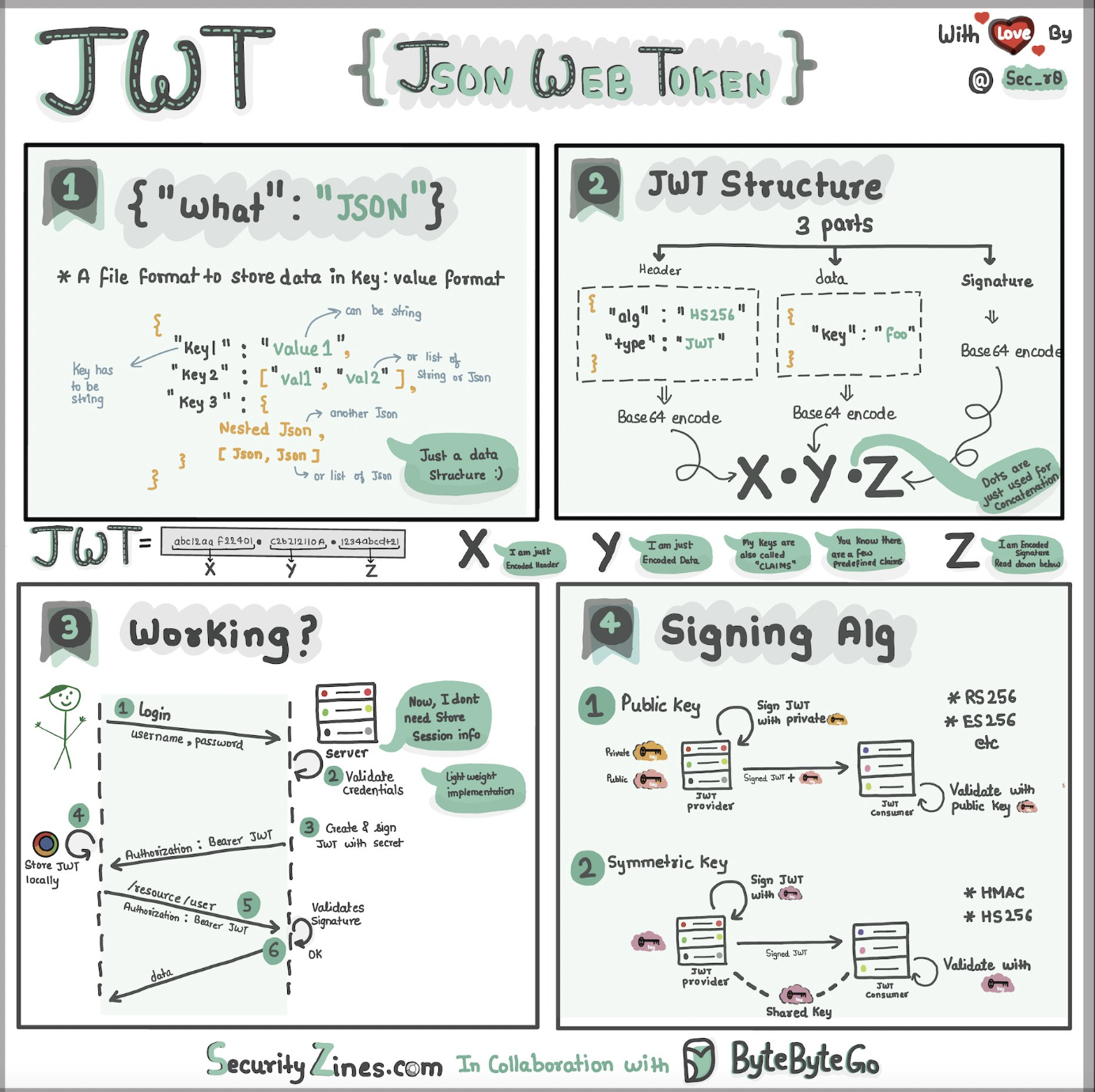
Rate limit vs. Throttle
Rate Limiting
- Definition: Rate limiting refers to the practice of restricting the number of requests a client can make to an API within a specific time period.
- Purpose: The primary purpose is to prevent abuse and protect the API from an excessive request load that could lead to resource exhaustion.
- Implementation: It is typically implemented using an approach such as “X requests per Y minutes”. For example, a rate limit might allow 1000 requests per hour.
- Response to Exceeding the Limit: When the limit is exceeded, the API typically responds with an error status, such as 429 Too Many Requests, and may include information about when the client can retry.
- Application: Rate limiting is often applied at the API key, IP address, or user account level.
Throttling
- Definition: Throttling refers to controlling the rate at which requests are processed by limiting the number of requests that can be processed in a given period of time.
- Objective: The primary objective is to ensure an equitable distribution of resources and to ensure that the system remains stable under high load without overloading the server.
- Implementation: It can be implemented using techniques such as “trickle” of requests to ensure that a constant number of requests are processed per unit of time.
- Response to Exceeding the Threshold: When the threshold is reached, additional requests may be queued or discarded, and clients may experience delays in response.
- Application: Throttling is often applied to control the overall load on the system and to manage the quality of service in real time.
Comparison
-
Time Focus:
- Rate Limiting: Based on fixed periods of time (e.g. 1000 requests per hour).
- Throttling: Based on the continuous processing rate (e.g., 10 requests per second).
-
Client Feedback:
- Rate Limiting: Typically returns an explicit error (429 Too Many Requests) when the limit is exceeded.
- Throttling: May cause requests to be delayed or queued, rather than returning an immediate error.
-
Control Objective:
- Rate Limiting: Prevent abuse and overuse by individual clients.
- Throttling: Maintain overall system stability and performance under varying loads.
-
Practical Examples
- Rate Limiting: A social networking API service may limit a user to posting a maximum of 100 tweets per hour to prevent spam.
- Throttling: A video streaming service may throttle the request rate to ensure that the server is not overloaded during peak hours, even if this means that some users may experience buffering.
In short, while both concepts aim to protect resources and maintain system performance, rate limiting is more focused on limiting the number of requests from a specific client in a given period of time, while throttling is more concerned with equitable distribution and maintaining system stability under high load.
Idempotency
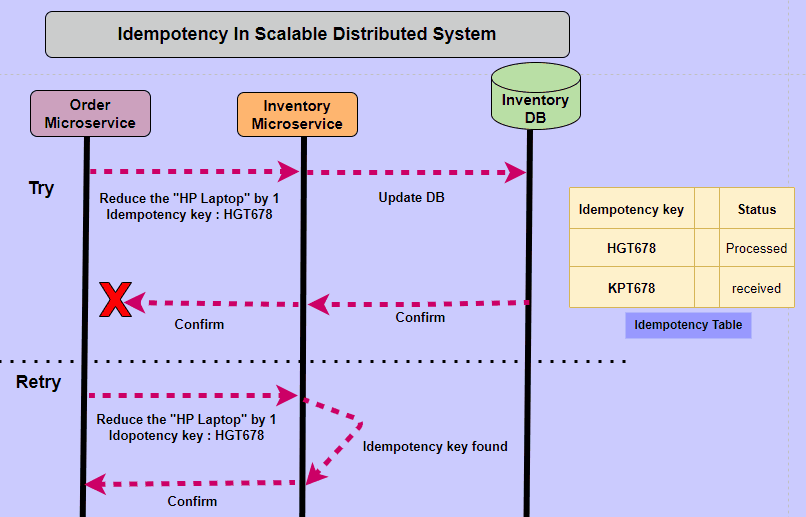
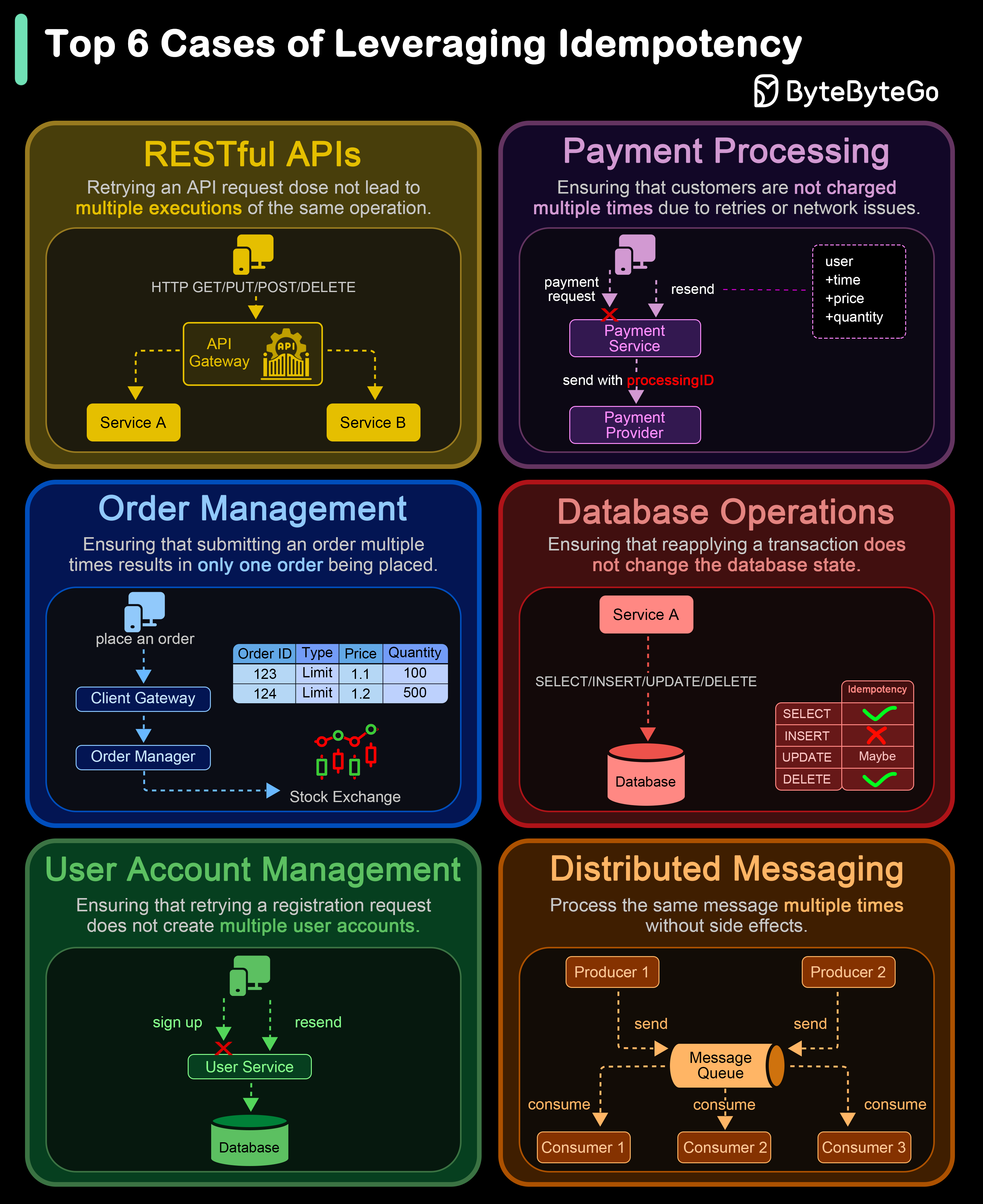
- Idempotent operations produce the same result even when the operation is repeated many times. The result of the 2nd, 3rd, and 1,000th repeat of the operation will return exactly the same result as the 1st time.
- Idempotency is a property of operations or API requests that ensures repeating the operation multiple times produces the same result as executing it once.
- Safe methods are idempotent but not all idempotent methods are safe.
- HTTP methods like GET, HEAD, PUT, DELETE, OPTIONS, and TRACE are idempotent, while POST and PATCH are generally non-idempotent.
- Understanding and leveraging the idempotent nature of HTTP methods helps create more consistent, reliable, and predictable web applications and APIs.
- Most HTTP methods used in REST APIs are idempotent, except for POST, and following REST principles can ensure proper usage of idempotent methods.
What is Idempotency?
-
Idempotency is a property of certain operations or API requests, which guarantees that performing the operation multiple times will yield the same result as if it was executed only once. This principle is especially vital in Distributed Systems and APIs, as it helps maintain consistency and predictability in situations such as network issues, request retries, or duplicated requests.
-
Idempotency is a crucial property of certain operations or API requests that guarantees consistent outcomes, regardless of the number of times an operation is performed. This principle simplifies error handling, concurrency management, debugging, and monitoring, while also enhancing the overall user experience.
-
For example, simple mathematical examples of idempotency include:
- x + 0;
- x = 5;
-
In the first example, adding zero will never change the result, regardless of how many times you do it.
-
In the second, x is always 5. Again, this is the case, regardless of how many times you perform the operation. Both of these examples describe an operation that is idempotent.
Why is Idempotency Important?
-
Idempotency is important in APIs because a resource may be called multiple times if the network is interrupted. In this scenario, non-idempotent operations can cause significant unintended side-effects by creating additional resources or changing them unexpectedly. When a business relies on the accuracy of its data, non-idempotency posts a significant risk.
-
Imagine a scenario where an API facilitates monetary transactions between accounts. A user sends a request to transfer $100 from Account A to Account B. Due to network latency or other factors, the request is unintentionally duplicated. Without idempotency, the API would process both requests, resulting in an unintended transfer of $200. However, if the operation is idempotent, only one transfer of $100 will occur, regardless of the number of duplicate requests.
-
Idempotency is crucial for ensuring the following:
- Consistency: The system maintains a predictable state, even when faced with request duplication or retries.
- Error Handling: Idempotent operations simplify error handling, as clients can safely retry requests without the risk of unintended side effects.
- Fault Tolerance: Idempotent APIs can better cope with network issues and other disruptions, ensuring a more reliable user experience.
Idempotent vs. Safe
-
The concepts of ‘idempotent methods’ and ‘safe methods’ are often confused. A safe method does not change the value that is returned, it reads – but it never writes. Going back to our previous examples:
- x + 0;
- x = 5;
-
The first of these, adding zero, will return the same value every time (it is idempotent), and adding zero will have no effect on that value (it is also safe). The second example will return the same value every time (it is idempotent) but is not safe (if x is anything other than 5 before the operation runs, it changes x).
-
Therefore, all safe methods are idempotent, but not all idempotent methods are safe.
Idempotent Methods in REST
- REST APIs use HTTP methods such as POST, PUT, and GET to interact with resources such as an image, customer name, or document.
- When using an idempotent method, the method can be called multiple times without changing the result.
- For example, using GET, an API can retrieve a REST resource.
- This has no effect on the resource and can be repeated over and over – it is both idempotent and safe.
HTTP Methods
- HTTP methods, or verbs, are the actions used to interact with resources in web-based systems, defining the type of operation a client wants to perform.
- Idempotency is an important property of some HTTP methods, ensuring that executing the same request multiple times produces the same result as if it was performed only once.
- GET, HEAD, PUT, DELETE, OPTIONS, and TRACE are idempotent methods, meaning they are safe to be retried or executed multiple times without causing unintended side effects.
- In contrast, POST and PATCH are generally considered non-idempotent, as their outcomes may vary with each request.
- Understanding and leveraging the idempotent nature of specific HTTP methods helps developers create more consistent, reliable, and predictable web applications and APIs.
POST
- POST is an HTTP (Hypertext Transfer Protocol) method used to submit data to a specified resource, typically for creating or updating content on a server.
- It is often employed when submitting form data, uploading files, or sending JSON payloads to an API.
- Unlike other HTTP methods like GET, PUT, or DELETE, POST is not inherently idempotent.
- This means that sending the same POST request multiple times can result in different outcomes or create multiple instances of the submitted data.
- Consequently, developers need to be cautious when handling POST requests, implementing mechanisms such as idempotency keys or other safeguards to prevent unintended side effects in cases where duplicate requests are submitted or retried.
GET
- The GET method is one of the fundamental HTTP methods used to request data from a specific resource on the web.
- When a client sends a GET request to a server, it is asking the server to retrieve and return the information associated with the specified resource, such as a webpage, image, or document.
- GET requests are designed to be read-only, meaning they should not modify the state of the resource or cause any side effects on the server.
- Due to their read-only nature, GET requests are considered idempotent. This means that making multiple identical GET requests to the same resource will yield the same result without causing any additional changes, ensuring consistency and predictability in Web-Based Applications And Services.
HEAD
- The HEAD method in HTTP is a request method used to retrieve metadata about a specific resource without actually downloading the resource’s content.
- Similar to the GET method, the HEAD method is used to request information about a resource, but it only returns the HTTP headers, which include information such as content type, content length, and last modification date.
- This method is particularly useful when clients want to check if a resource exists or retrieve its metadata without incurring the cost of downloading the entire resource.
- The HEAD method is idempotent, meaning that multiple requests using HEAD on the same resource will yield the same outcome without any side effects.
- Consequently, it is safe to perform HEAD requests multiple times without affecting the state of the resource or the server.
PUT
- PUT is an HTTP method used to update or replace an existing resource on the server with new data provided by the client. The request includes a unique identifier, such as a URI, which the server uses to locate the target resource.
- The client also provides a payload, containing the updated data to be applied to the resource. As an HTTP method, PUT is inherently idempotent.
- This means that making multiple identical PUT requests will have the same effect as making a single request. If a resource already exists at the specified URI, the server will replace it with the new data. If the resource does not exist, the server may create it, depending on the implementation.
- The idempotent nature of PUT ensures consistency and predictability in the face of network issues or duplicate requests, making it well-suited for updating resources in a distributed system.
PATCH
- PATCH is an HTTP method used for partially updating resources on a server. Unlike PUT, which typically requires the client to send a complete representation of the resource, PATCH allows clients to send only the changes that need to be applied.
- This makes it more efficient for updating specific attributes or elements of a resource without affecting the rest of the data. The idempotency of PATCH depends on the implementation and the semantics of the operation.
- While PATCH can be idempotent if the changes are applied in a consistent and deterministic manner, it is not inherently idempotent like GET, PUT, and DELETE.
- In practice, PATCH can be made idempotent by carefully designing the API and the underlying operations, ensuring that repeated PATCH requests yield the same outcome as a single request.
DELETE
- DELETE is an HTTP method used to remove a specified resource from a server. It is part of the HTTP/1.1 standard and allows clients to request the deletion of a resource identified by a specific Uniform Resource Identifier (URI).
- When a DELETE request is successfully processed, the server typically responds with a 204 No Content status code, indicating that the resource has been removed. The DELETE method is considered idempotent, as performing the same request multiple times results in the same outcome.
- After the initial successful deletion, any subsequent DELETE requests to the same URI should have no additional effect on the server’s state, since the resource is no longer present.
- This idempotent nature ensures consistency and predictability, making the DELETE method a reliable choice for managing resource deletion in APIs and web services.
TRACE
- TRACE is an HTTP method that allows clients to retrieve a diagnostic representation of the request and response messages for a specific resource on the server. When a client sends a TRACE request, the server returns the entire HTTP request, including headers, as the response body.
- This method is primarily used for debugging purposes, enabling developers to examine and diagnose potential issues in the request and response cycle.
- TRACE is considered idempotent because, by design, it does not alter the resource or its state on the server.
- When issuing multiple TRACE requests, the server will consistently return the same request data, making it a safe and predictable operation.
- However, due to security concerns, TRACE is often disabled on web servers to prevent potential information leaks.
- As can be seen from this information, most of the methods used in a REST API are idempotent.
- The exception is POST. However, it is worth remembering that if used incorrectly, other methods like GET and HEAD can still be Called In A Non-Idempotent Way. Developers can avoid this by following REST principles.
POST vs PUT vs PATCH
- Use POST to create new resources on collection endpoints, such as api.library.com/books.
- Use PUT to update resources on entity endpoints, such as api.library.com/books/iliada.
- Use PATCH to partially update resources, also on entity endpoints.